Have you ever wondered how apps on your phone can store and access data such as notes, user preferences or other important information? There are several ways that app developers often use, especially in iOS app development, such as User Default, Core Data, File System, SQLite, Keychain, Firebase Database or even Realm.
You could say that all these forms are data persistence. However, did you know that at WWDC 2023, Apple announced its latest data persistence? The answer is SwiftData, a new data persistence designed to be easier to write declaratively with SwiftUI. Wow, interesting, isn’t it? Let’s talk about it in more detail.
SwiftData is Apple’s new framework to persist information from our app. This framework allows us to create a database inside our app and be able to save, query, update and delete information. It is completely designed for SwiftUI but it can be decoupled from the UI.
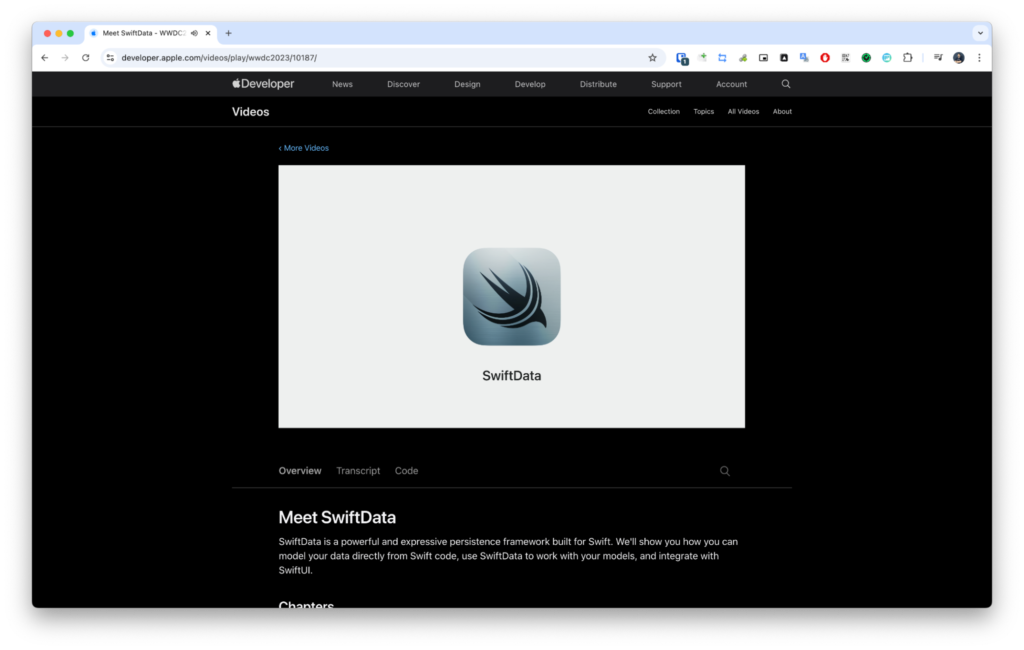
Data Persistence
Before we get into what SwiftData is, are you familiar with the term data persistence? This term refers to when an application has the ability to store data so that it can be retrieved and used later, even if the application is opened or closed. Therefore, data persistence ensures that data remains intact and can be used later.
So what exactly is the difference between each data persistence in iOS app development? Let’s discuss the differences first.
User defaults: For storing simple data such as application configuration in the form of key-value pairs. Limited to small and simple data types.
Core Data: Framework used to manage complex data and features object graph management and data persistence. Suitable for applications with complex data structures.
File System: Manually stores data in the form of files (e.g. JSON, plist, images) in the application directory. Flexible, but does not automatically manage data like Core Data.
SQLite: A lightweight relational database that allows SQL queries. Suitable for applications that require full control over the database structure and relationships between data.
Keychain: For storing sensitive data such as passwords and authentication tokens with high security. Data is stored in encrypted form.
Realm: A high-performance, easy-to-use mobile database similar to Core Data, but simpler and faster in terms of querying and data management.
There are many methods for implementing data persistence in iOS applications. Of course, each of them has advantages and disadvantages. You can use them according to your needs and conditions.
For example, when you want to store sensitive information, Keychain may be a recommendation. Another example is when you want your data to be accessible to other operating systems, Firebase Database may be the one to choose.
Core Data as integrated data persistence in Xcode
You should also know that implementing various data persistence methods is easy to do, especially in UIKit-based application development.
Usually, it is only necessary to create a separate class to manage the mechanism by which data is stored so that it can be reused. For example, Core Data is the default data persistence in Xcode.
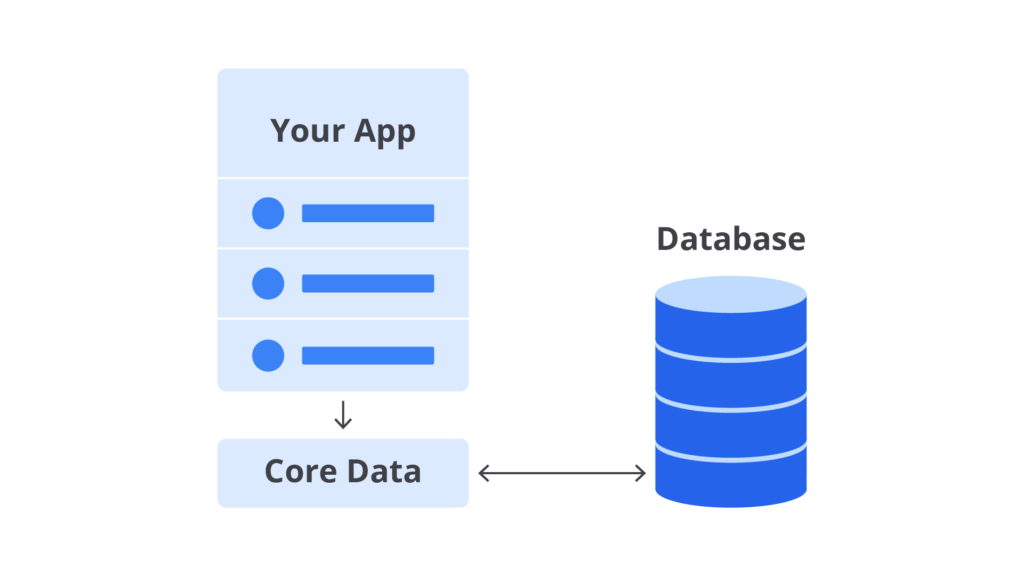
It seems quite easy to implement Core Data in a UIKit application. However, it is necessary to know that each data persistence has its own way of implementation. For example, Core Data has a Core Data Stack that contains a collection of framework objects as part of the Core Data initialisation.
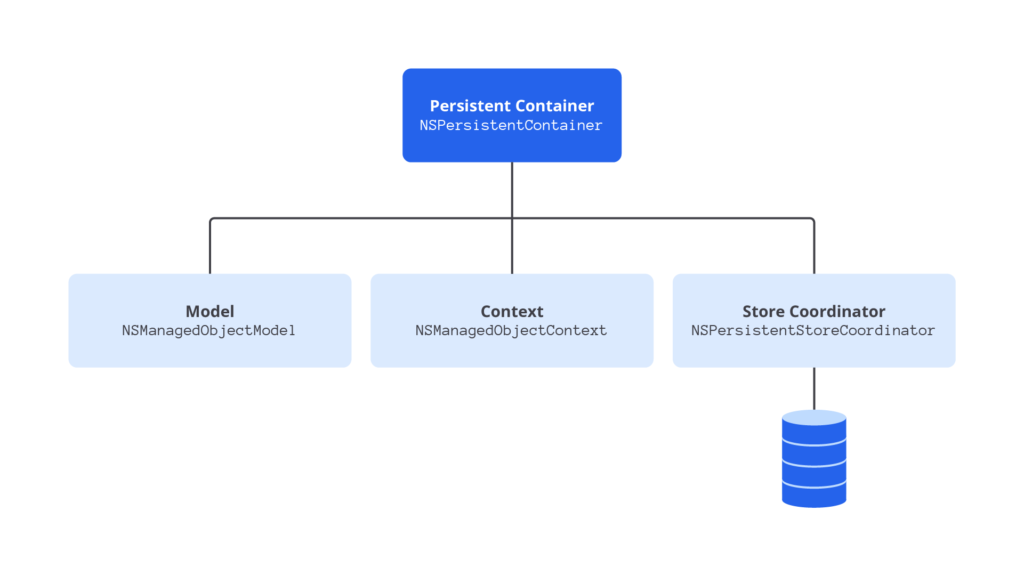
It is necessary to use some of these supporting frameworks for Core Data to work optimally. The complexity increases because Core Data is designed with an imperative programming paradigm.
Especially when using SwiftUI, you have to adjust some parts of your code to make the integration with SwiftUI, which is based on declarative programming, work well. As a result, you will experience many problems when using Core Data in SwiftUI applications. In fact, not many developers have switched from Core Data to other persistence data, such as Realm. Therefore, Apple announced a suitable persistence data for SwiftUI, SwiftData.
SwiftData, Apple’s new data persistence
After Apple developers had problems with the implementation of Core Data in SwiftUI, Apple announced SwiftData at WWDC 2023. SwiftData is a data persistence that makes it easier to develop iOS apps, especially SwiftUI, because it uses declarative code. You can query and filter using Swift code and easily integrate it with SwiftUI.
SwiftData Features
Models with Swift
You can convert Swift models to SwiftData models. You can do this by adding @Model to the beginning of the model class. Simple, isn’t it?
@Model
class Recipe {
@Attribute(.unique) var name: String
var summary: String?
var ingredients: [Ingredient]
}